JUnit-Server: Effizientes Testen von Backend-Anwendungen
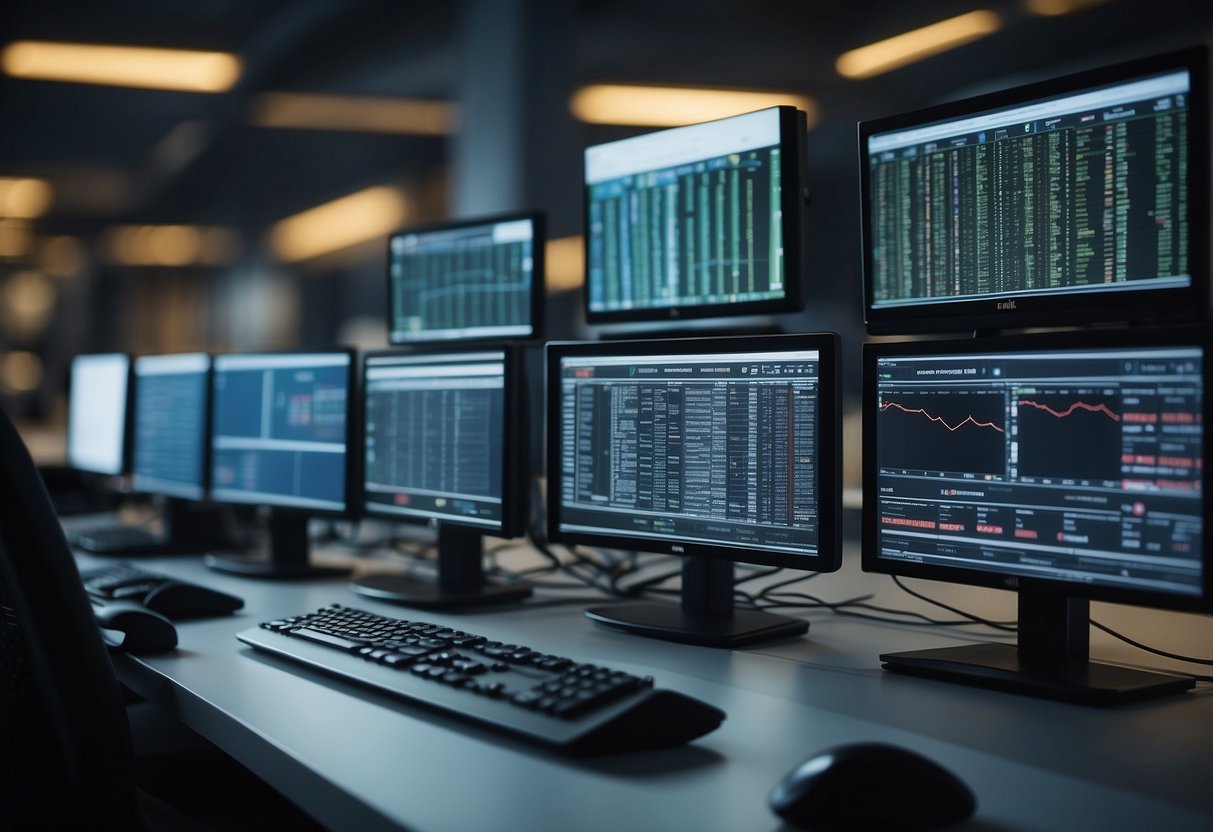
JUnit-Servers ist eine einfach zu verwendende Bibliothek, die ermöglicht, Ihre Webanwendung innerhalb eines Servlet-Containers zu testen. Wenn Sie JUnit Jupiter verwenden, lässt sich dies durch eine benutzerdefinierte Erweiterung bewerkstelligen. Auch für JUnit 4 gibt es einfache Lösungen, wie die Verwendung eines benutzerdefinierten JUnit Runners oder einer JUnit Regel.
Diese Tools sind besonders nützlich, wenn es darum geht, die Funktionalität Ihrer Serverlogik sicherzustellen. Mit JUnit-Servers können Sie HTTP-Anfragen an Ihren Server senden und die Antworten mit den erwarteten Ergebnissen abgleichen. Diese Art von automatisiertem Testen spart Ihnen nicht nur Zeit, sondern verbessert auch die Zuverlässigkeit Ihrer Anwendungen deutlich.
Wenn Sie JUnit noch nicht ausprobiert haben, bietet Ihnen die umfangreiche Dokumentation einen umfassenden Leitfaden. Dadurch werden Sie nicht allein beim Schreiben von Tests unterstützt, sondern erhalten auch wertvolle Informationen über Erweiterungen und Anpassungsmöglichkeiten.
Key Takeaways
- JUnit-Servers ermöglicht das Testen von Webanwendungen in einem Servlet-Container.
- Es bietet einfache Lösungen sowohl für JUnit Jupiter als auch für JUnit 4.
- Automatisiertes Testen verbessert die Zuverlässigkeit Ihrer Anwendungen.
JUnit Server-Integration
Bei der Integration eines JUnit-Servers spielen mehrere wichtige Faktoren eine Rolle. Zunächst sind die Grundlagen entscheidend, gefolgt von der Konfiguration und schließlich den Erweiterungen und Plugins, die zur Verfügung stehen.
Grundlagen der JUnit Server
JUnit-Server bieten eine praktische Möglichkeit, Webanwendungen innerhalb eines Tests auszuführen. Diese Server kommen oft als embedded Versionen wie Jetty und Tomcat. Sie ermöglichen es Ihnen, Ihre Webanwendung in einer kontrollierten Umgebung zu testen.
Mit JUnit 4 und 5 können Sie spezielle JUnit-Rules oder Extensions verwenden, um den Server zu starten und zu stoppen. Dies führt zu besseren und konsistenteren Testergebnissen.
Aufbau und Konfiguration
Der Aufbau eines JUnit-Servers beginnt mit dem Einfügen der nötigen Maven-Abhängigkeiten in Ihr Projekt. Beispiele sind:
<dependency> <groupId>com.github.mjeanroy</groupId> <artifactId>junit-servers</artifactId> <version>1.0.0</version> </dependency>
Dann müssen Sie den Server konfigurieren. Stellen Sie sicher, dass Sie die korrekten Ports und Einstellungen verwenden. Ein Beispiel:
HttpServer httpServer = HttpServer.create(new InetSocketAddress(8000), 0);
Dadurch können Sie Mockserver und Mockserver-Client-Java ebenfalls leicht integrieren.
Erweiterungen und Plugins
Für die Erweiterung der Funktionen stehen mehrere Plugins zur Verfügung. Das Mockserver Maven Plugin ist beliebt für das Mocken von HTTP-Requests:
<plugin> <groupId>org.mock-server</groupId> <artifactId>mockserver-maven-plugin</artifactId> <version>5.11.2</version> </plugin>
Zusätzlich gibt es Erweiterungen für Spock und Mockito, die Integrationstests erleichtern. Diese Plugins helfen Ihnen, Ihre Tests zu automatisieren und zu verbessern und sind oft im zentralen Maven-Repository zu finden.
Die Wahl der richtigen JUnit-Version (4 oder 5) und der benötigten Java APIs ist ebenfalls entscheidend, um ein optimales Testergebnis zu erzielen.
Praktische Anwendung von JUnit Servern
JUnit Server bieten eine effektive Möglichkeit, Webanwendungen in einer kontrollierten Umgebung zu testen. Du kannst bestimmte Tests durchführen, erweiterte Konzepte anwenden und die Überwachung und das Log-Management effektiv verwalten.
Tests mit JUnit Server
Mit JUnit-Servern kannst du HTTP-Server wie com.sun.net.httpserver.HttpServer einrichten, um verschiedene Aspekte deiner Anwendung zu testen. Diese Server können auf einem spezifischen Port laufen und erlauben es, eingehende Anfragen über Methoden wie GET, POST usw. zu testen.
Du kannst @Test-Annotationen verwenden, um Testfälle zu definieren. Beispielsweise, um die Antwort auf eine Anfrage zu überprüfen, die mit einem bestimmten JSON-Schema oder regulären Ausdrücken übereinstimmt. Die Headers der Anfrage können leicht überprüft und angepasst werden.
Beispiel-Tabelle:
Funktion Beschreibung
| @Test | Markiert JUnit-Testmethoden
| Port | Definiert den Kommunikationsport
| Headers | Überprüft und manipuliert Anfrage-Header
Erweiterte Konzepte
Erweiterte Konzepte wie Custom Rules und Callback-Mechanismen bieten zusätzliche Flexibilität beim Testen. Du kannst Request Matcher verwenden, um bestimmte Anfragen anhand von Regulären Ausdrücken oder JSON-Schema zu identifizieren. Dies hilft dir, spezifische Szenarien präzise zu testen.
Mit VerificationTimes kannst du sicherstellen, dass bestimmte Anfragen eine vorgegebene Anzahl an Aufrufen erreicht haben. Bei Fehlern kannst du spezifische Fehlerlogs generieren und die Ursprünge der Fehler leicht nachverfolgen.
Erweiterte Sicherheitskonzepte wie Secure-Verbindungen können ebenfalls getestet werden, um die Integrität und Sicherheit deiner Anwendung sicherzustellen.
Überwachung und Log-Management
Eine effektive Überwachung und Verwaltung der Logs ist entscheidend, um die Leistung und Sicherheit deiner Anwendung im Blick zu behalten. Mithilfe von Logs kannst du die Details jeder Anfrage und Antwort einsehen, was beim Debugging und Optimieren hilfreich ist.
Regelmäßige Überwachung hilft, potentielle Probleme frühzeitig zu erkennen. Die Verwendung von Tools zur Überwachung von JVM-Leistungsdaten, zum Beispiel, kann wertvolle Einblicke bieten. Ein gut konfiguriertes Log-Management-System unterstützt dich dabei, Anomalien und sicherheitsrelevante Vorfälle zu überwachen und schnell zu reagieren.
Die Kombination dieser Konzepte hilft, robuste und zuverlässige Tests durchzuführen und die Wartbarkeit deiner Anwendung zu erhöhen.
Frequently Asked Questions
Dieser Abschnitt behandelt häufig gestellte Fragen zu JUnit. Sie erfahren, wie Sie Test Suiten erstellen, Annotationen verwenden, JUnit mit Gradle integrieren und vieles mehr.
Wie kann ich eine JUnit Test Suite erstellen?
Um eine JUnit Test Suite zu erstellen, nutzen Sie die Annotation @RunWith(Suite.class) und die @Suite.SuiteClasses-Annotation, um die zugehörigen Testklassen anzugeben.
Wie verwendet man Annotationen in JUnit?
In JUnit nutzen Sie Annotationen wie @Test, @Before, und @After, um bestimmte Methoden als Testmethoden zu kennzeichnen und um Setup- und Teardown-Methoden zu definieren.
Wie integriere ich JUnit mit Gradle?
Um JUnit mit Gradle zu integrieren, fügen Sie testImplementation 'junit:junit:Version' in Ihre build.gradle-Datei ein. Ersetzen Sie Version durch die gewünschte JUnit-Version.
Wie nutzt man MethodSource in JUnit für parametrisierte Tests?
Mit @MethodSource können Sie in JUnit 5 parametrisierte Tests schreiben. Definieren Sie eine statische Methode, die die Input-Parameter zurückgibt und verknüpfen Sie diese mit der Testmethode.
Muss man in JUnit 5 Testmethoden öffentlich deklarieren?
Nein, in JUnit 5 müssen Testmethoden nicht öffentlich sein. Sie können den Standard-Zugriffsmodifikator (package-private) verwenden.
Was ist der Unterschied zwischen JUnit 4 und JUnit 5?
JUnit 5 bietet mehrere Verbesserungen gegenüber JUnit 4, wie die Unterstützung von Java 8, eine verbesserte Erweiterbarkeit, und die Trennung von Jupiter, Platform und Vintage Modulen.
Neue Beiträge
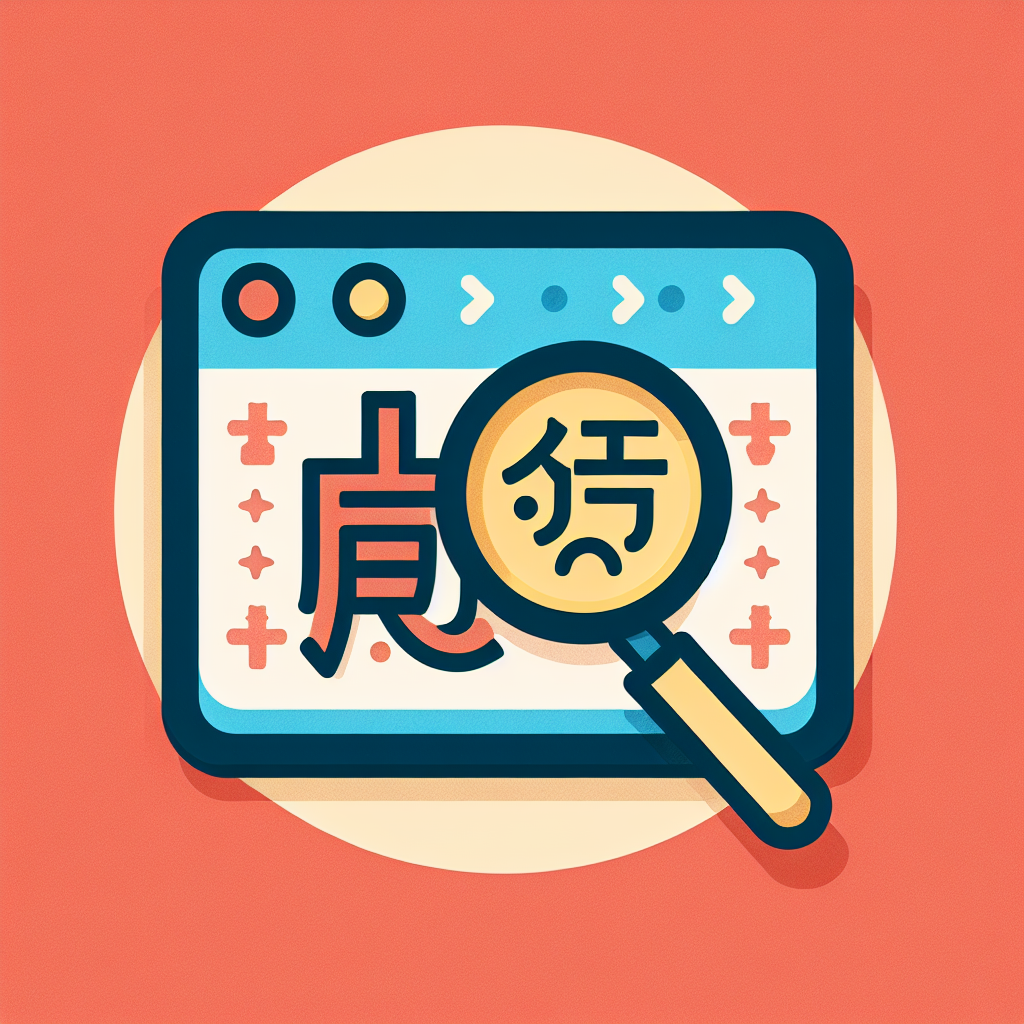
Alpha Zeichen zum Kopieren: Alles, was du wissen musst
Wissenschaft
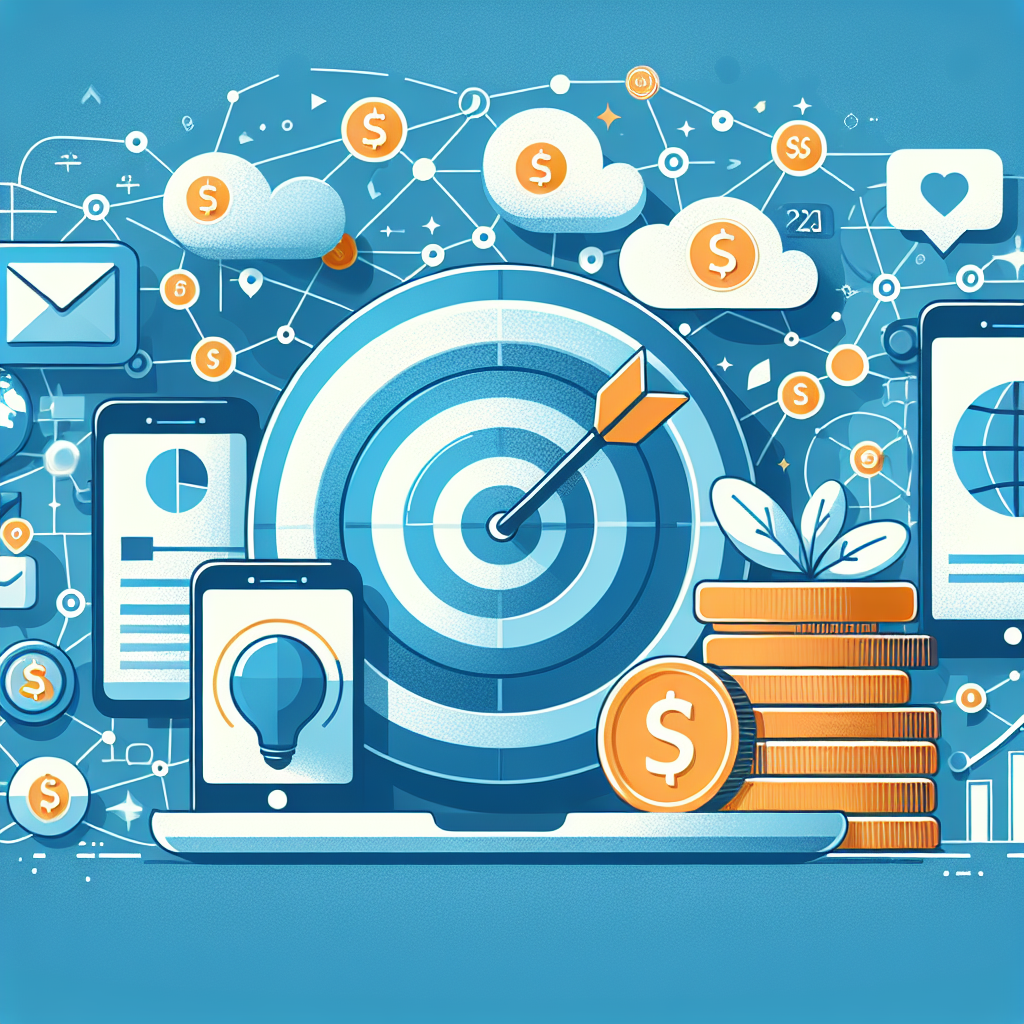
Technische Symbole: Bedeutung und Anwendung in der Praxis
Technik
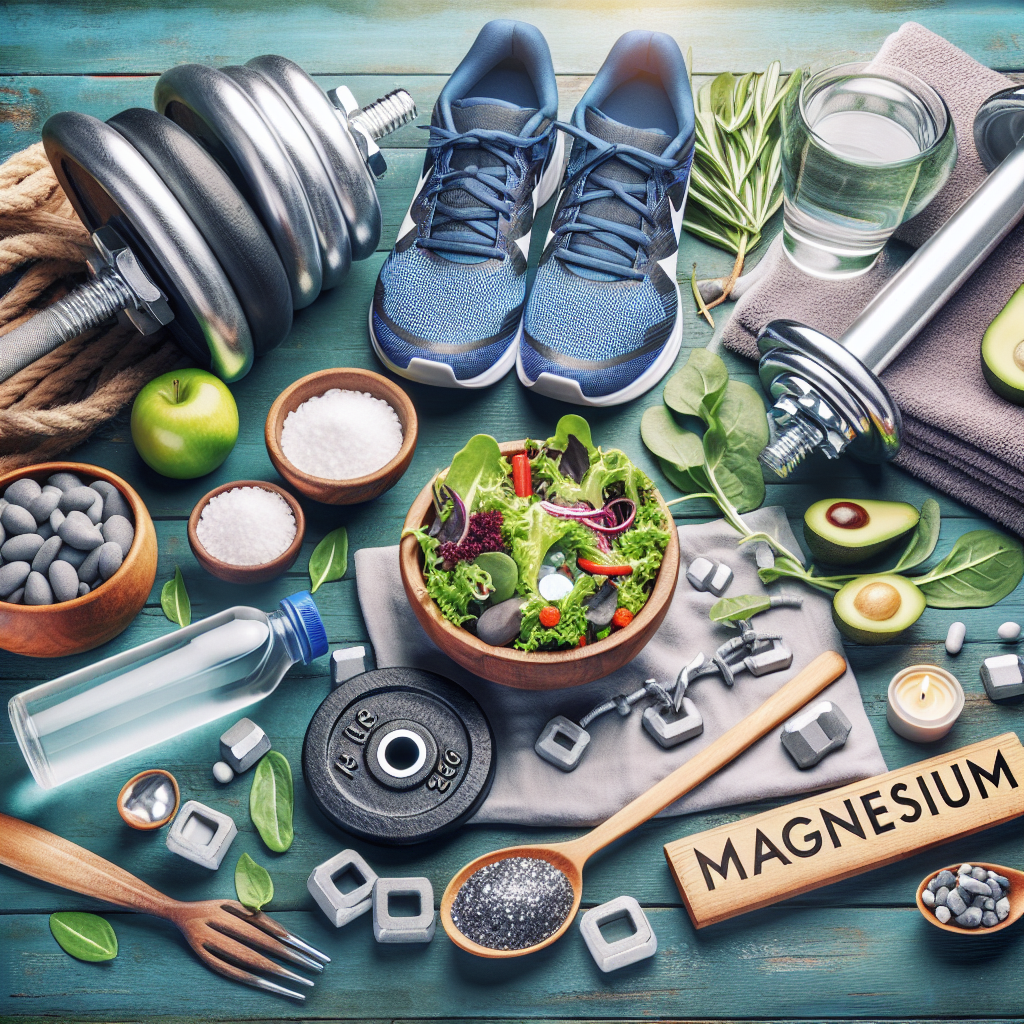
Die Funktionsstruktur nach VDI 2221: Ein tiefgehender Einblick
Ingenieurwesen

Eine Anleitung zum Kopieren japanischer Zahlen
Reisen
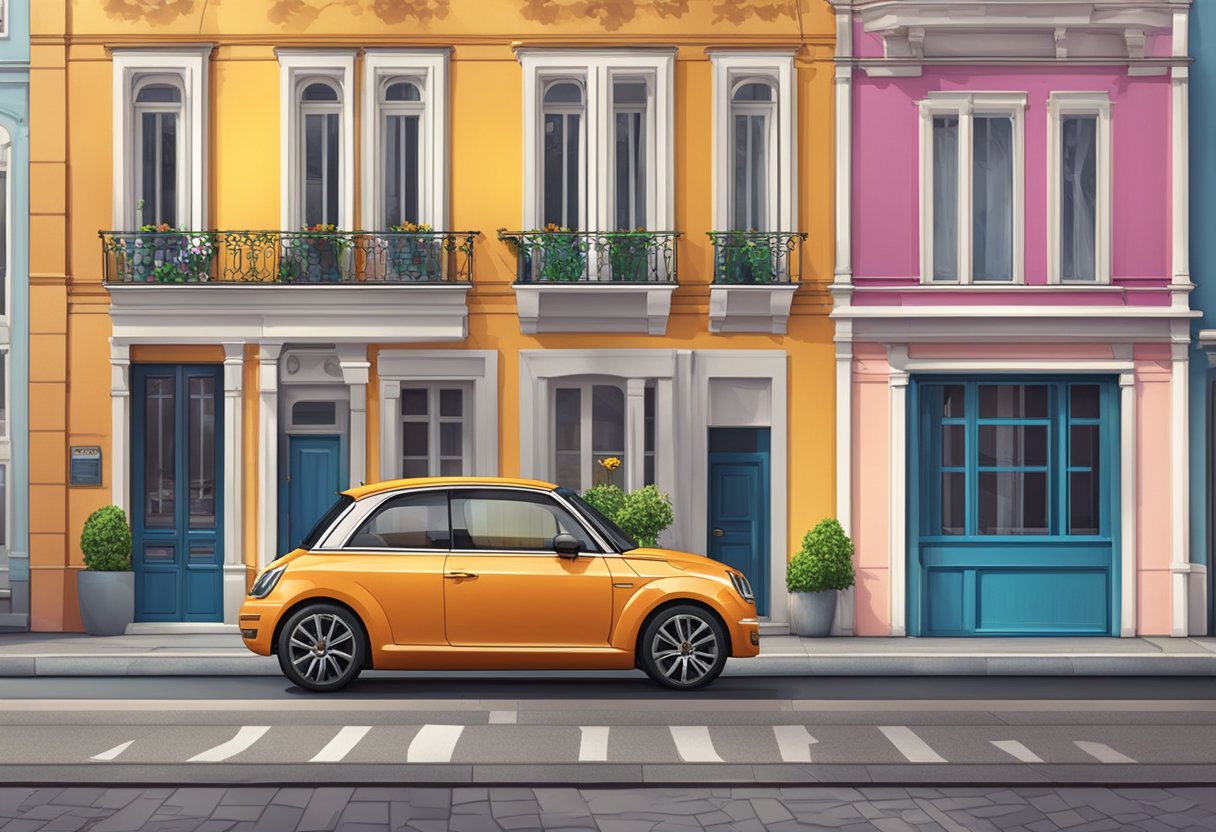
Die besten Tastenkombinationen für das Alpha-Zeichen
Technologie
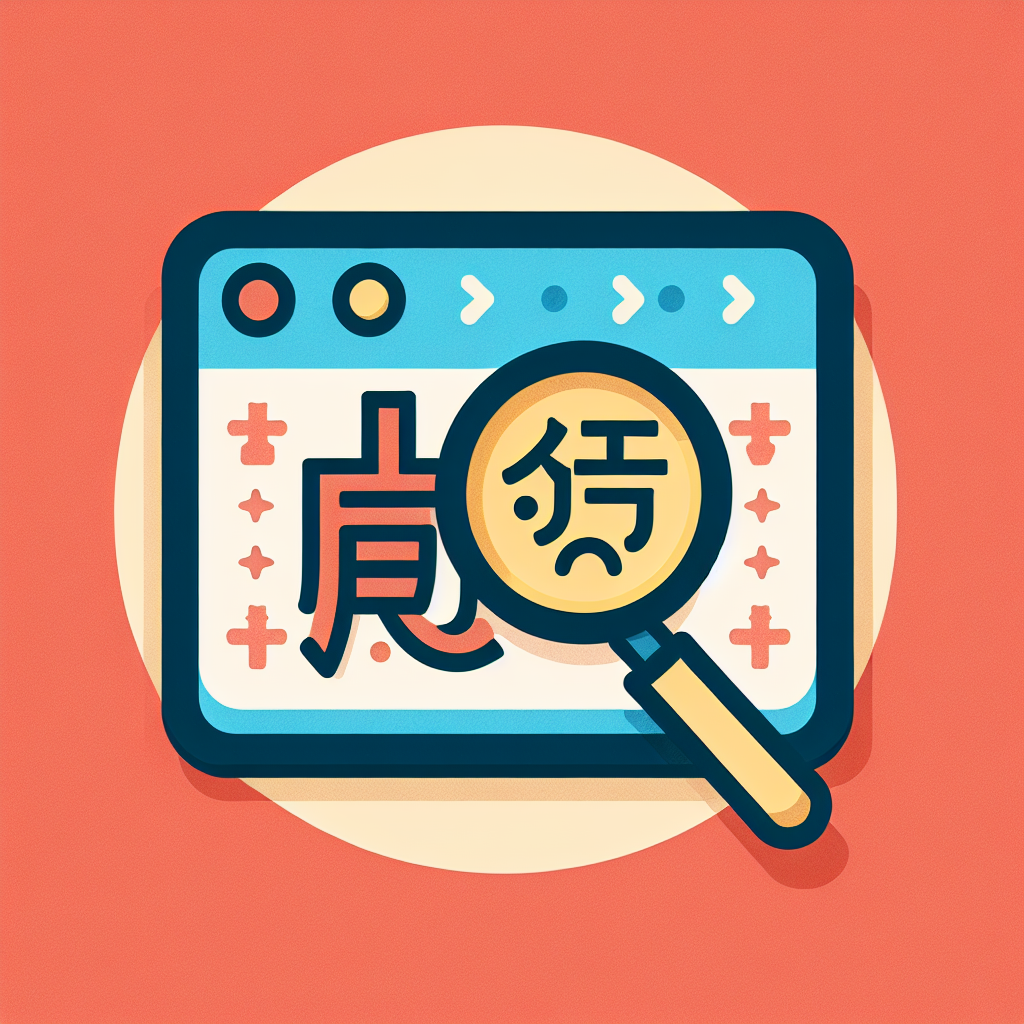
Die Rolle der Zeichensprache in Technischen Plänen
Technik
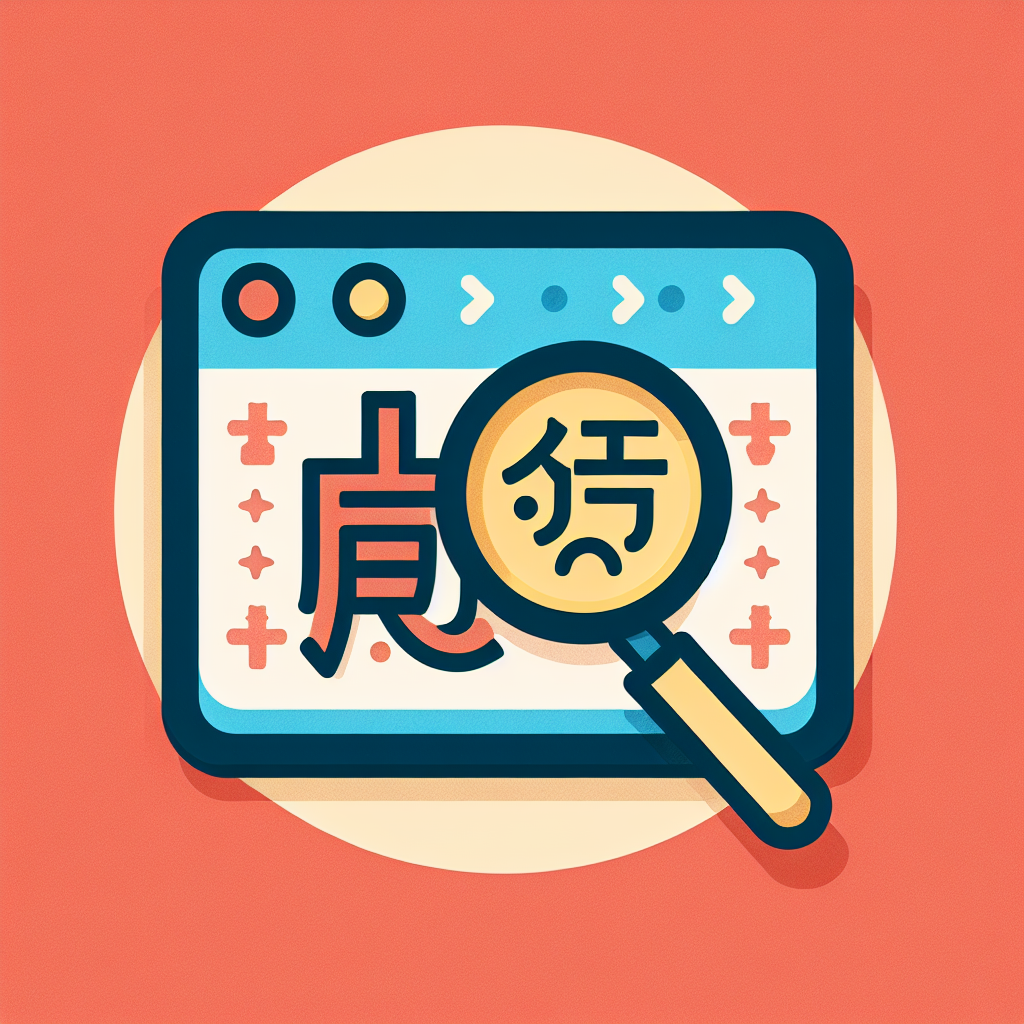
Griechische Buchstaben zum Kopieren: Der perfekte Leitfaden
Mathematik
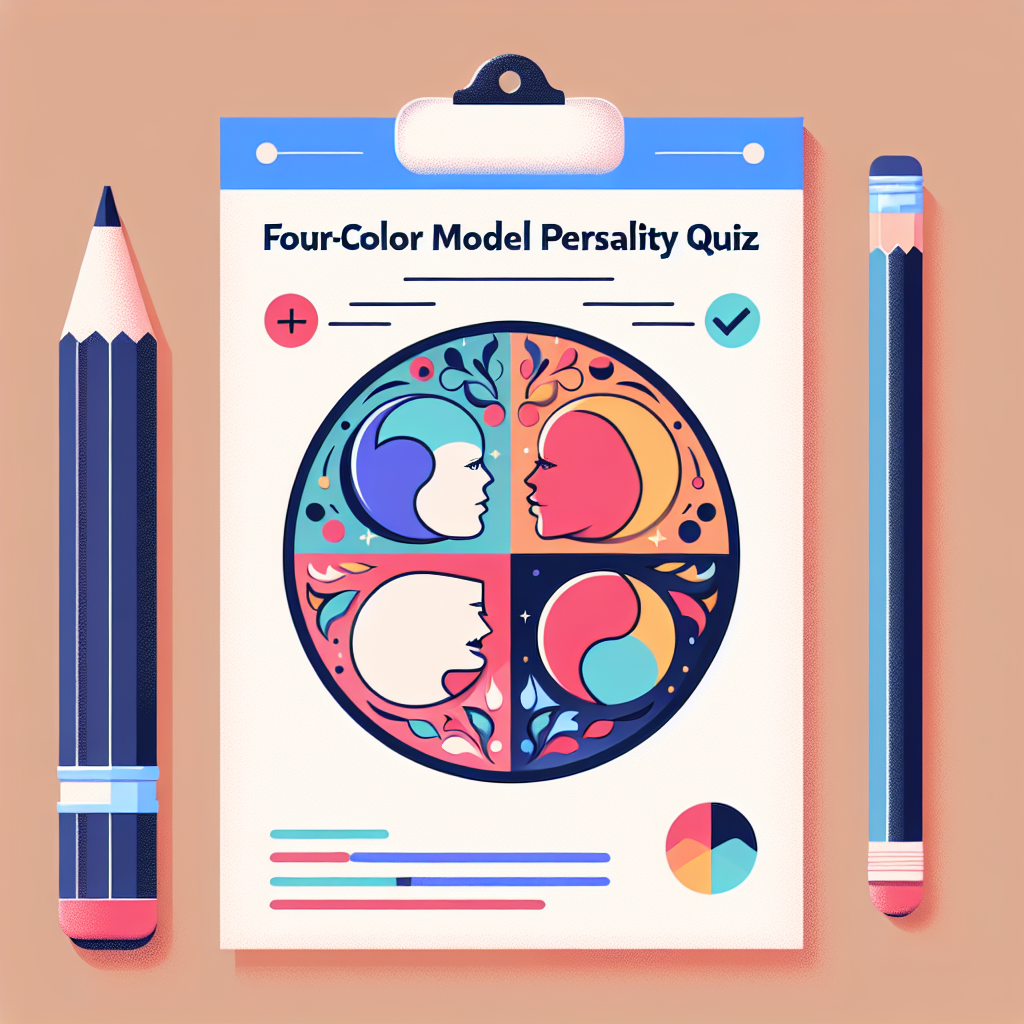
Symbole Elektrotechnik PDF: Alles, was Sie wissen müssen
Technik
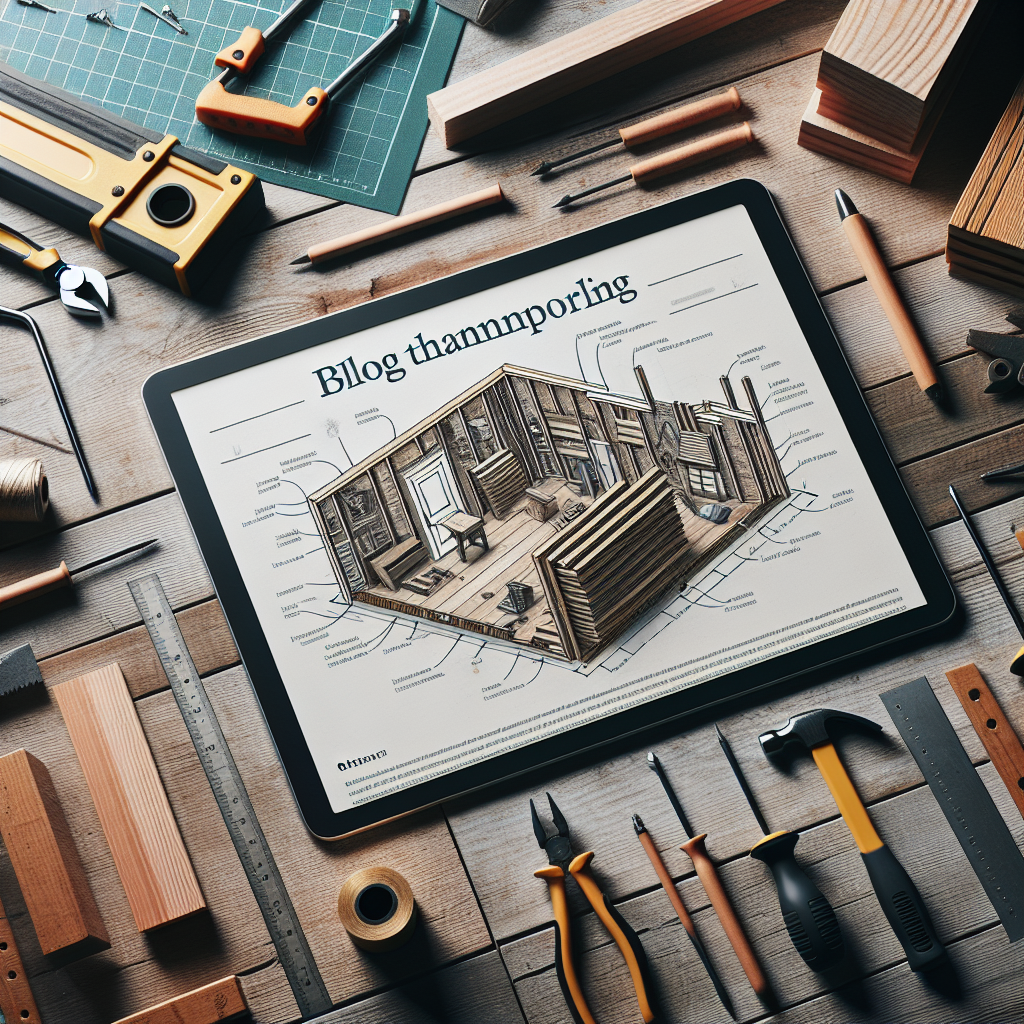
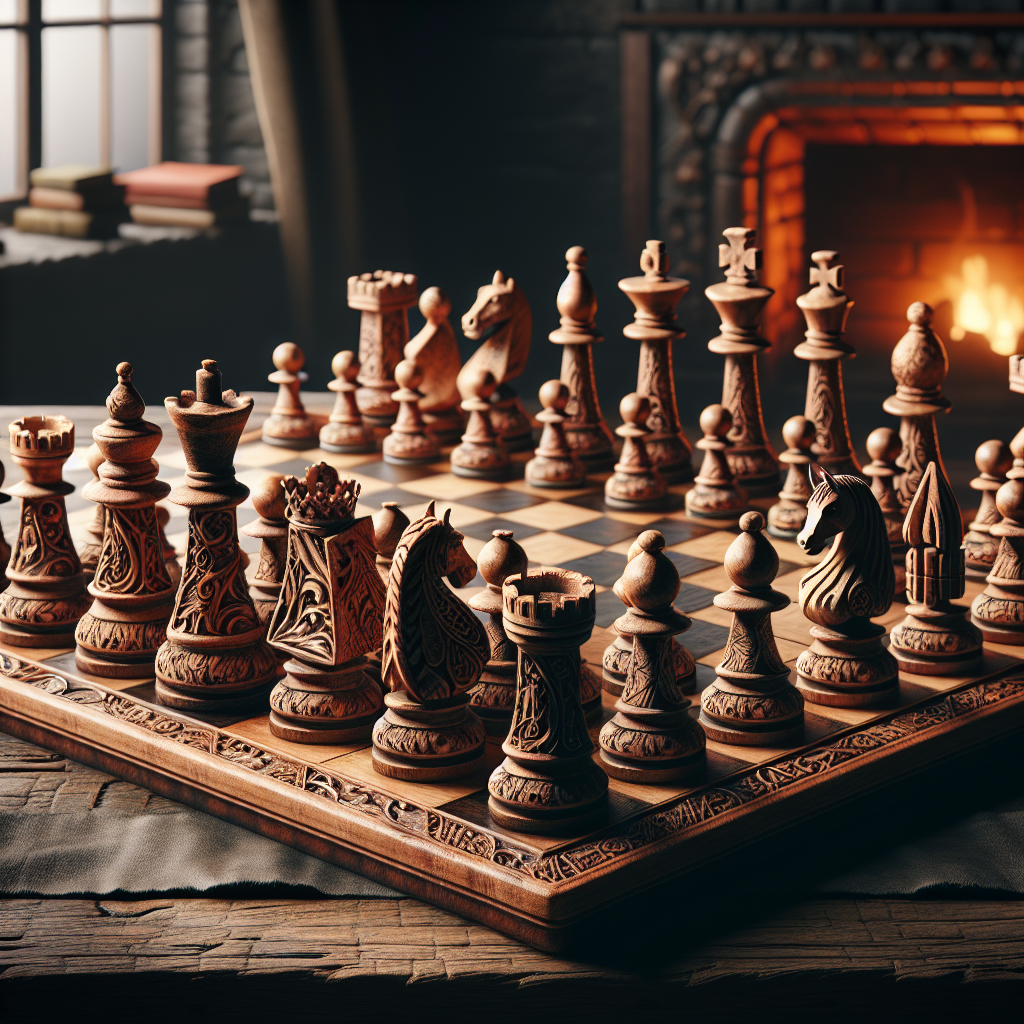
Die tiefere Bedeutung der japanischen Kalligrafie
Kunst
Beliebte Beiträge
Japanische Schriftzeichen zum Kopieren: Eine Anleitung für die Verwendung von Kanji und Katakana
Einführung in Japanische Schriftzeichen
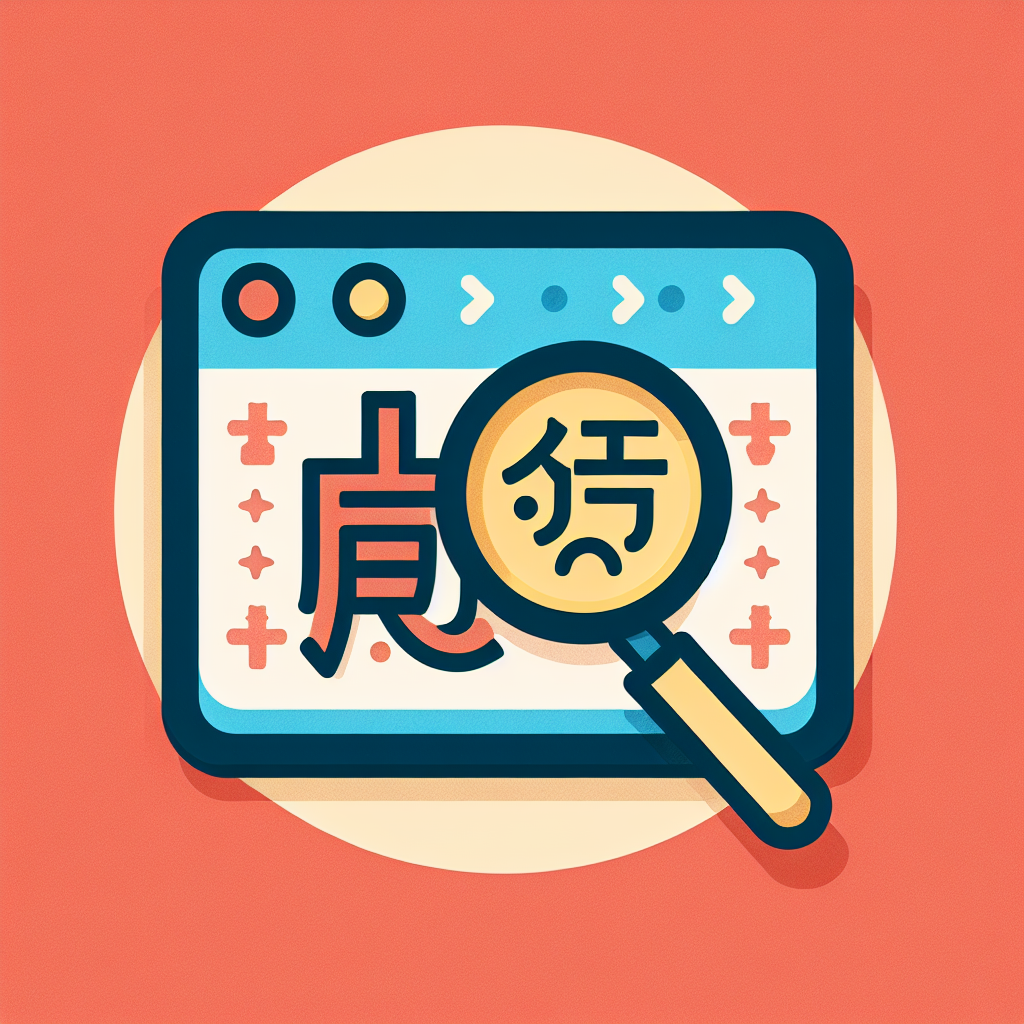
Schöne Zahlen zum Kopieren: Dein Guide für ästhetische Ziffern
Social Media
Zahlen zum Kopieren: Wo Sie kostenlose Zahlensymbole finden können
Produktivität
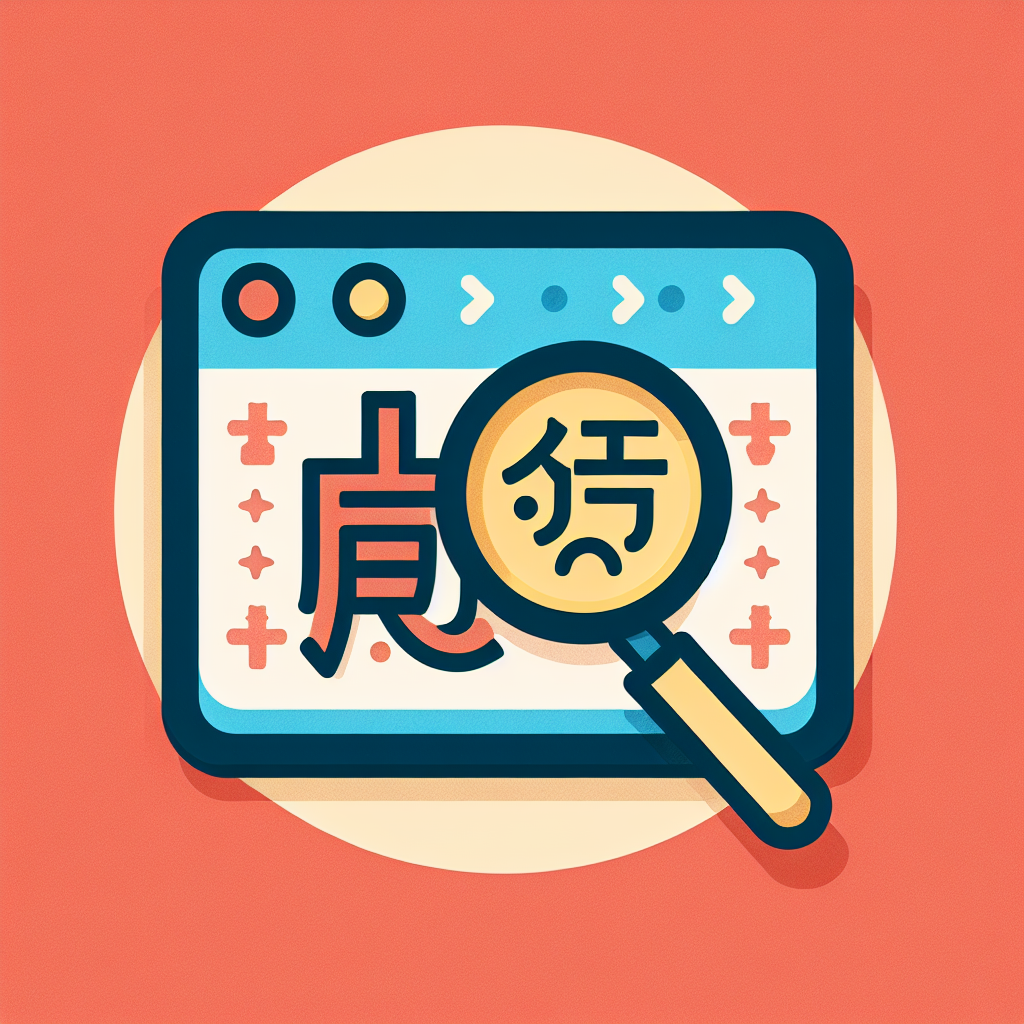
Noten Symbole zum Kopieren: So findest und nutzt du sie effektiv
Musik
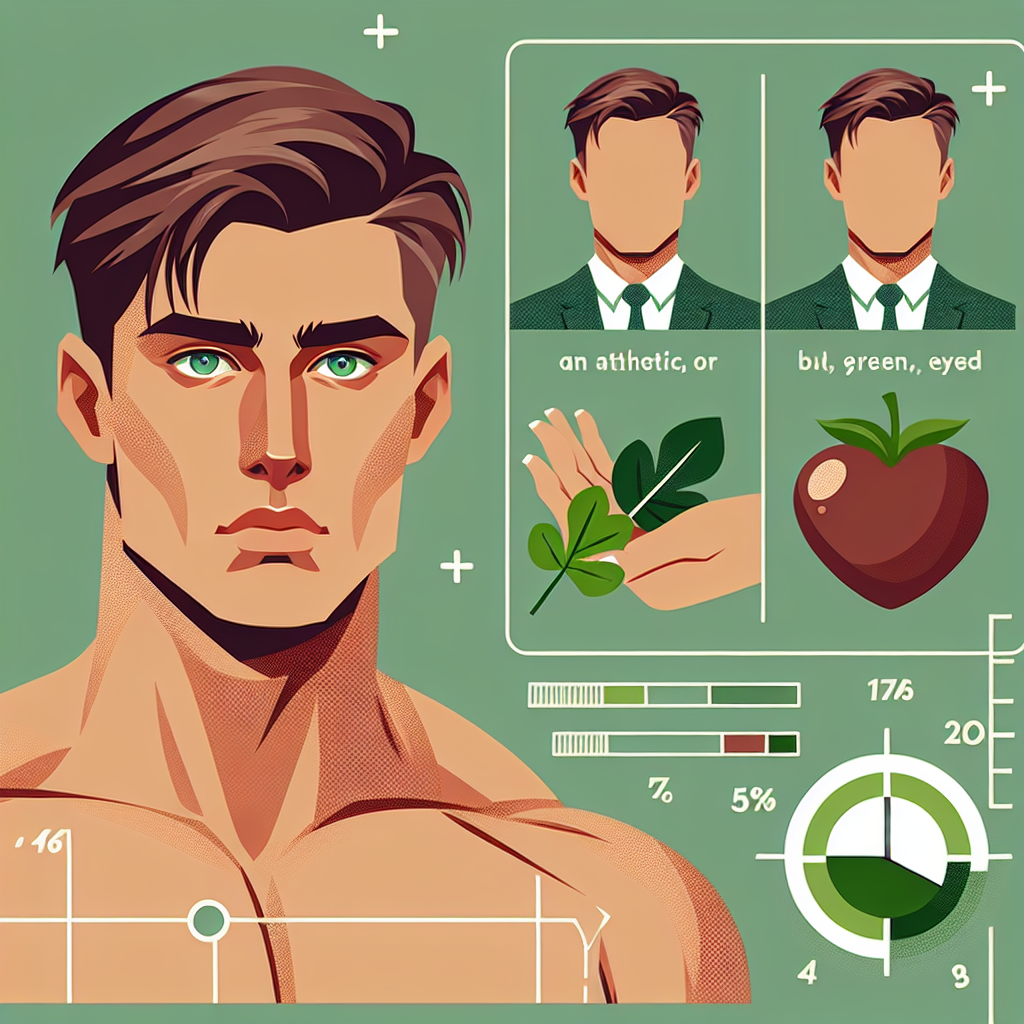
Die Bedeutung und Verwendung von Sonderzeichen in der digitalen Kommunikation
Technologie
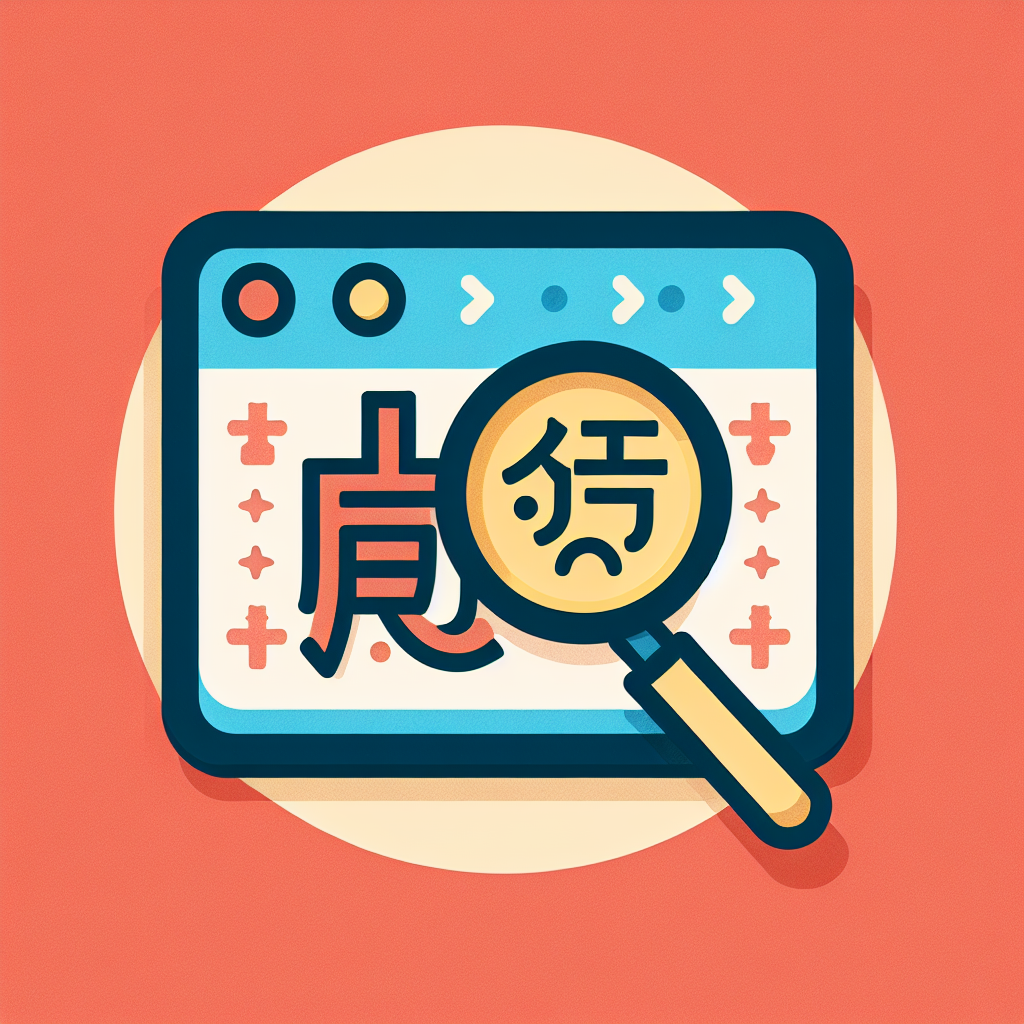
Chinesische Zeichen zum Kopieren: So findest und nutzt du sie effektiv
Kultur
Rechteck Symbol: Bedeutung und Verwendung in der Grafikdesign-Industrie
Grafikdesign
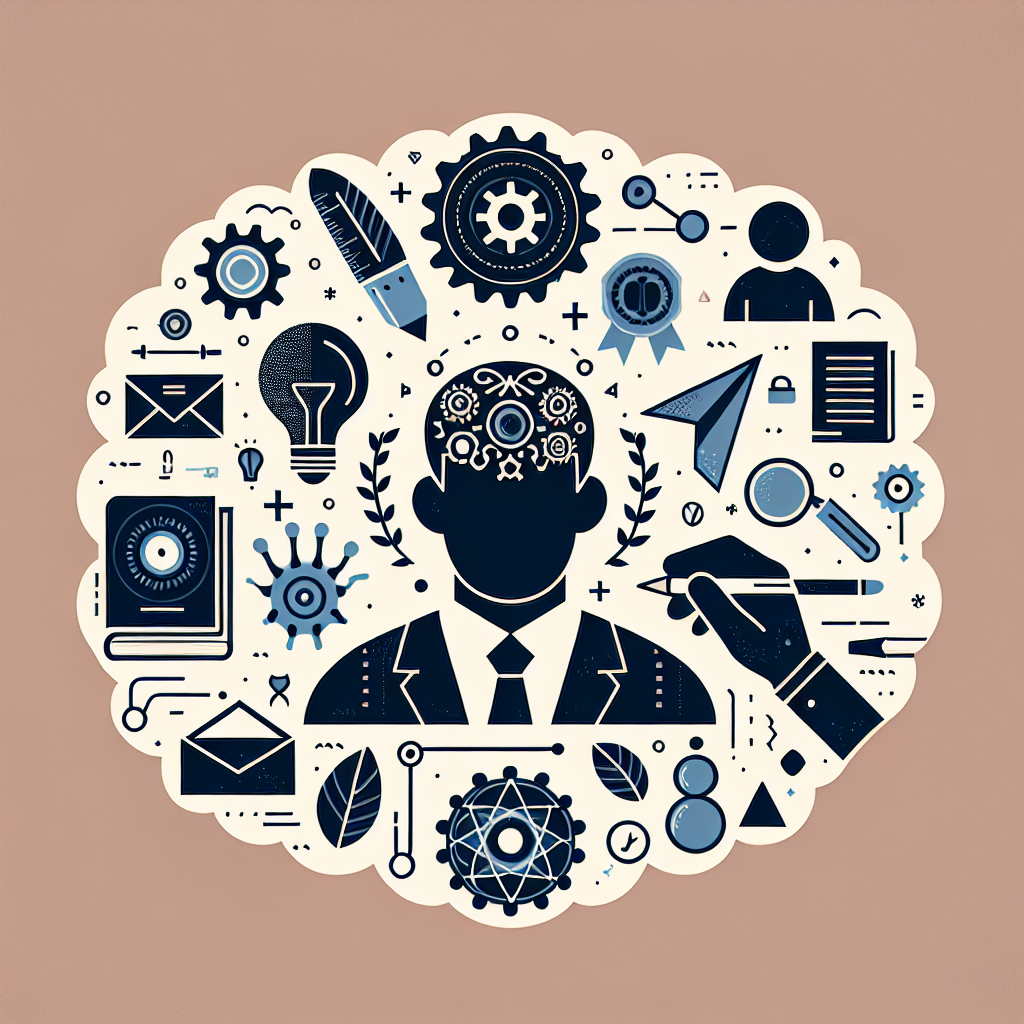
Wellenende DIN 748: Spezifikationen, Anwendungen und Vorteile
Maschinenbau
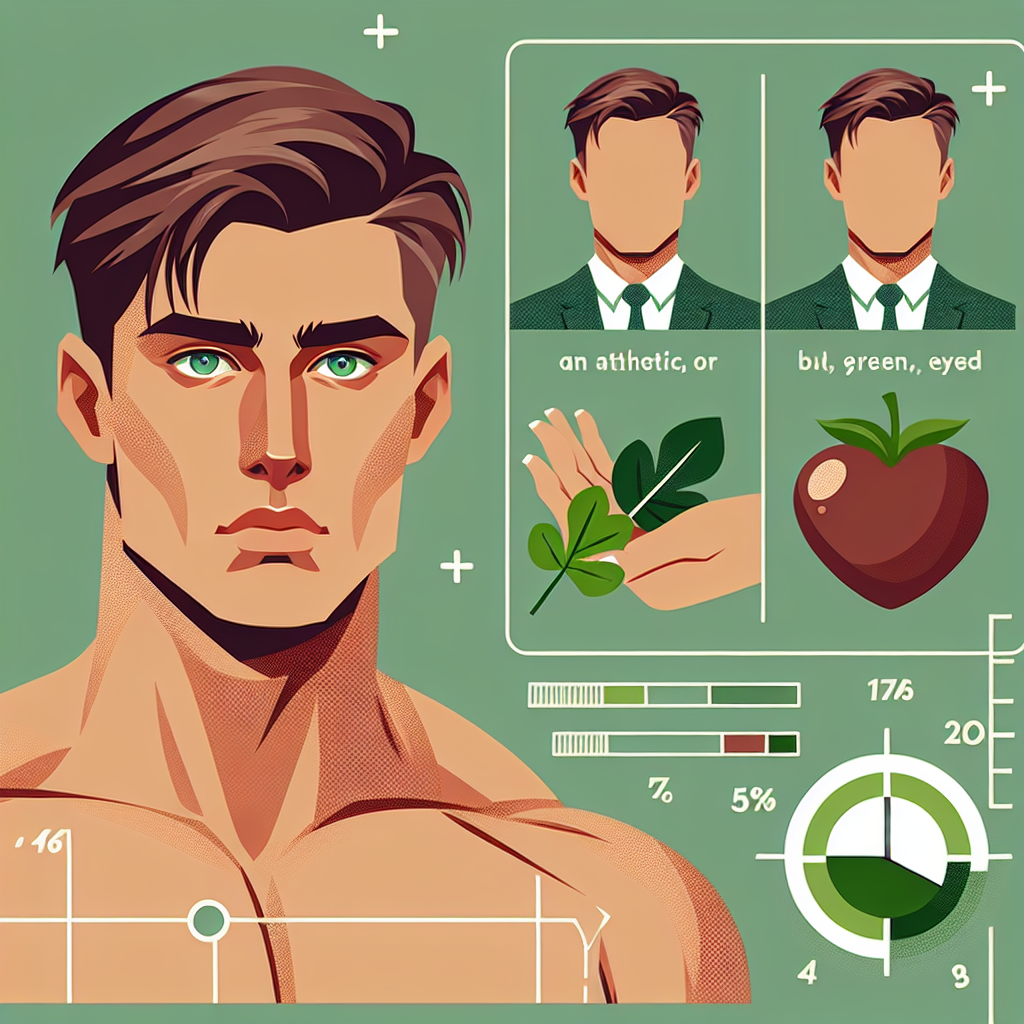
Arten von Datenbanken: Ein Überblick über die verschiedenen Typen und ihre Anwendungen
Technologie
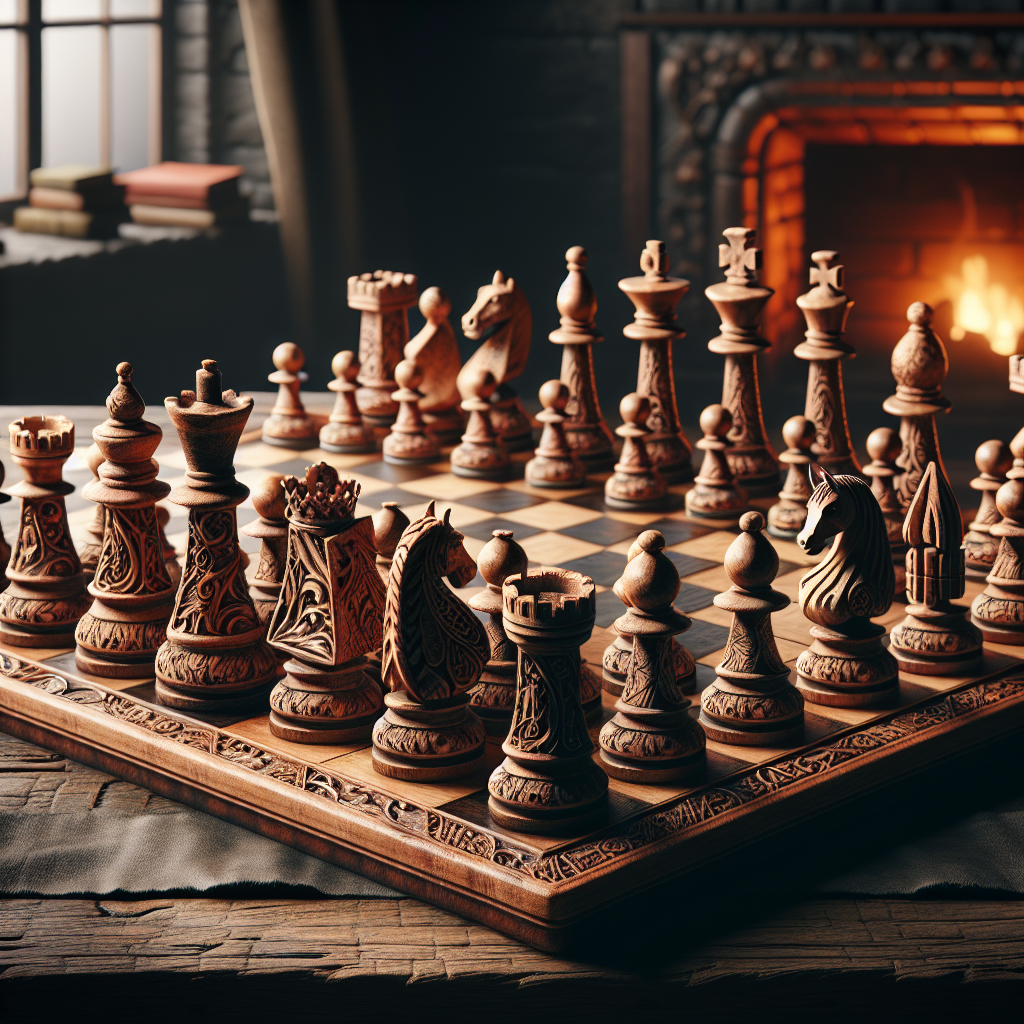
Schach Symbole: Alles, was Sie über die Bedeutung der Schachfiguren wissen müssen
Schachfiguren und deren Bedeutung